Changing Images
Using onclick
in HTML to change an image source
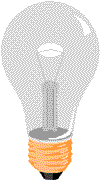

Changing Style
Using onclick
in HTML to style an HTML element
Example: onclick="document.getElementById('demo').style.visibility='hidden'"
Strings
Note: Strings are immutable. All string methods return a new string.
Examples: l = myString.length();
s = myString.slice(0, 5);
user = inputData.toUpperCase();
.length()
returns the length of a string.
.slice(start, end)
returns a part of a string.
.replace("text", "replacement")
replaces the first found value with another value.
.toUpperCase()
converts a string to upper case.
.toLowerCase()
converts a string to lower case.
.concat("string1", " ", "string2")
concatenates a string to another string (or set of strings).
.trim()
removes whitespace from the beginning and the end of a string.
.trimStart()
and .trimEnd()
can also be used.
.charAt(index)
returns the character at a specified index
.split(" ")
converts the parts separated by the specified character in a string into an array.
String Search Methods
Replace "x" with the value to search for. If not found, the index returned will be -1.
.indexOf("x")
, .lastIndexOf("x")
, and .search("x")
return the index of the occurrence of a string.
.match("x")
returns an array of the matching results.
.includes("x")
returns true if it contains the specified string.
.startsWith("x")
returns true if it begins with the specified string.
.endsWith("x")
returns true if it ends with the specified string.
Numbers
Note: if the argument is not a number, NaN (not a number) will be returned.
Examples: n = myNumber.toString();
dob = Number(userInput)
avg = parseFloat("3.15 average");
.toString()
returns a number as a string.
Number(var)
converts a variable or a date to a number.
parseInt(string)
parses a string and returns the first integer.
parseFloat(string)
parses a string and returns the first number.
Number.isInteger(num)
returns true if the argument is an integer.
Loops
Loops using for
iterate a set number of times using a counter
Loops using while
iterate until a condition is met
Loops using do
... while
iterate at least once
Loops using for
... of
iterate through elements of an array or characters of a string
Loops using for
... in
iterate through keys of an object
The break
keyword can be used to get out of a loop
The continue
keyword can be used to skip to the next iteration
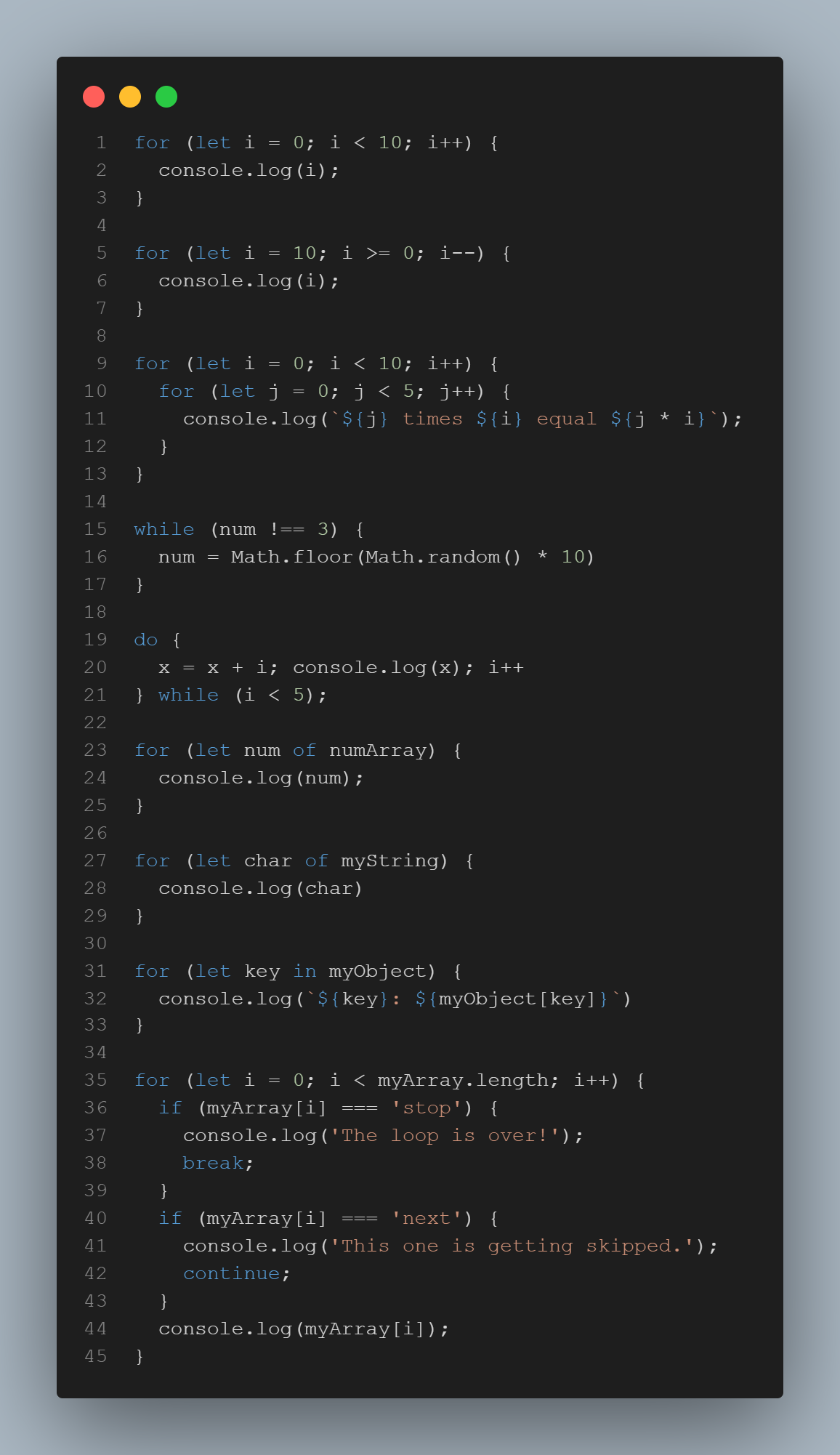
Functions
A function is a reusable block of code that groups together a sequence of statements to perform a specific task.
A parameter is a variable which will be assigned the value of the argument passed in when the function is called.
Default parameters allow us to assign a default value to a parameter in case no argument is passed into the function.
To return a value from a function, we use a return statement.
A helper function is a function called by another function.
A function expression is stored in a variable.
An anonymous function is a function with no name.
The arrow function syntax is a shorter way to write functions by using the special “fat arrow” () =>
notation.
A consise arrow notation is a condensed form of an arrow function. One parameter does not need to be enclosed in parentheses, but no parameter or more than one will need parentheses. If the function's body has a single-line block, it does not need curly braces and the return keyword can be omitted (implicit return).
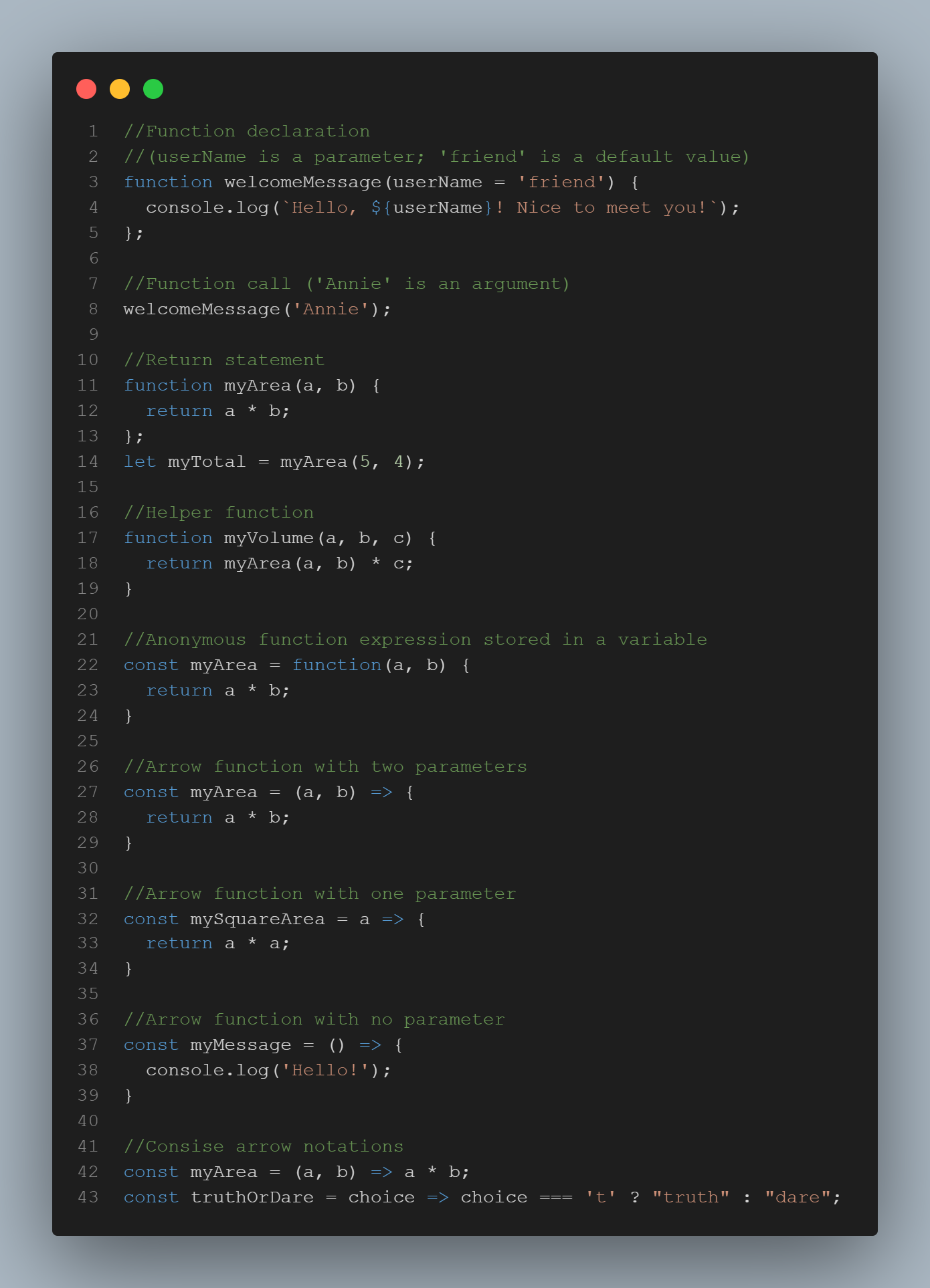
Arrays
Array Methods tbat converts an Array to a String (or vice-versa)
.toString()
converts the array to a string.
.join(" ")
converts the array to a string separated by the character(s) specified.
Array.from("string")
creates an array from a string.
Array Methods that Mutate the Array
.pop()
removes the last item and returns that item.
.push(element)
adds an item at the end and returns the new length.
.shift()
removes the first item and returns that item.
.unshift(myElement)
adds an item at the beginning and returns the new length.
.sort()
sorts alphabetically.
.reverse()
reverses the elements.
.splice(start, removed, myElement)
adds and/or remove elements and returns the removed elements.
Array Methods that Return a New Array
.slice(start, end)
creates a new array with removed elements.
.concat(array2)
concatenates an array to another array and returns the result.
.flat()
Combines all the arrays within the array into one single array.
.map(myFunction)
creates a new array by performing a function on each element.
Example: smallNumbers = myNumbers.map(num => { return num / 100 })
.filter(myFunction)
creates a new array with elements that pass a test.
Example: smallNumbers = myNumbers.filter(num => { return num < 250 })
Methods to Search an Array
.indexOf(myElement)
returns the index of an element.
.includes(myElement)
returns true if the element is present.
.find(myFunction)
returns the value of the first element that passes the test.
Example: smallNum = myNumbers.find(num => { return num < 10 })
.findIndex(myFunction)
returns the index of the first element that passes the test.
Example: smallNumIndex = myNumbers.findIndex(num => { return num < 10 })
Other Array Methods
.length()
returns the size of the array.
.forEach(myFunction)
calls a function for each element.
Example: myNumbers.forEach(num => { console.log(`Number ${num}`) })
.reduce(myFunction)
runs a function on each element to produce a single value.
Example: mySum = myNumbers.reduce((accum, num) => { return accum + num }, 10)
.every(myFunction)
returns true if all values pass the test.
Example: smallNum = myNumbers.every(num => { return num < 10 })
.some(myFunction)
returns true if some of the values pass the test.
Example: smallNum = myNumbers.some(num => { return num < 10 })
Objects
An object is a built-in data type for storing key-value pairs.
Either .
or [key]
is used to access or change the value of a key-value pair or add a new one.
The keyword delete
deletes both the key and the value.
Object methods are property values that are functions.
Destructuring assignment is used to extract object properties into variables.
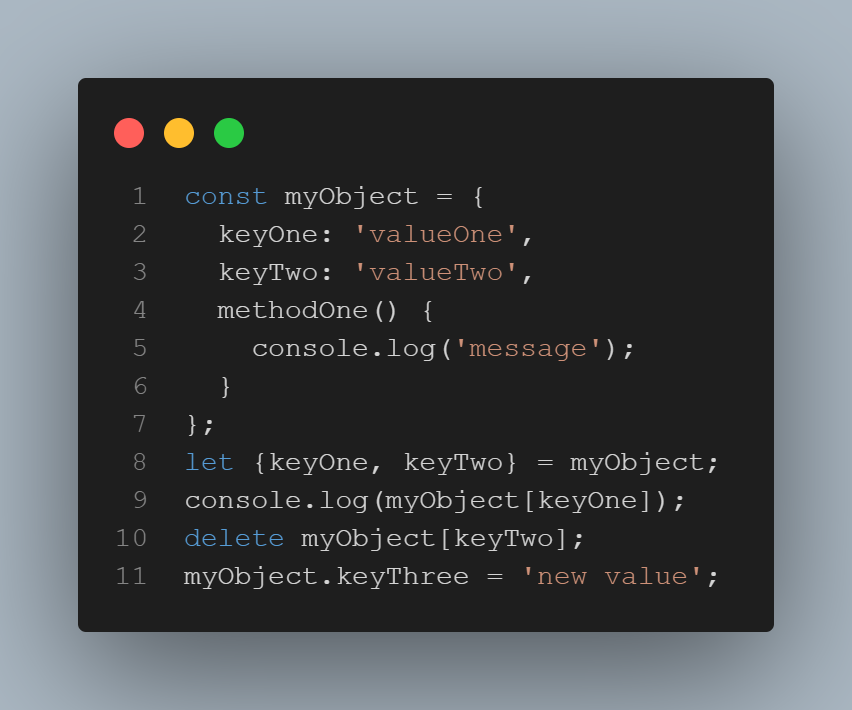
Classes
A class is a template for creating objects.
By convention, we capitalize and PascalCase class names.
A setter is a method to set a new value using the keyword set
.
A getter is a method to get an existing value using the keyword get
.
Methods in classes do not have any separators between them.
JavaScript calls the constructor
method every time it creates a new instance.
New instances are created using the new
keyword.
static
methods are called on the class itself.
A child class can extend a parent class by using the extends
keyword.
A child class constructor calls the parent class constructor using the super
method. super
must be called first.
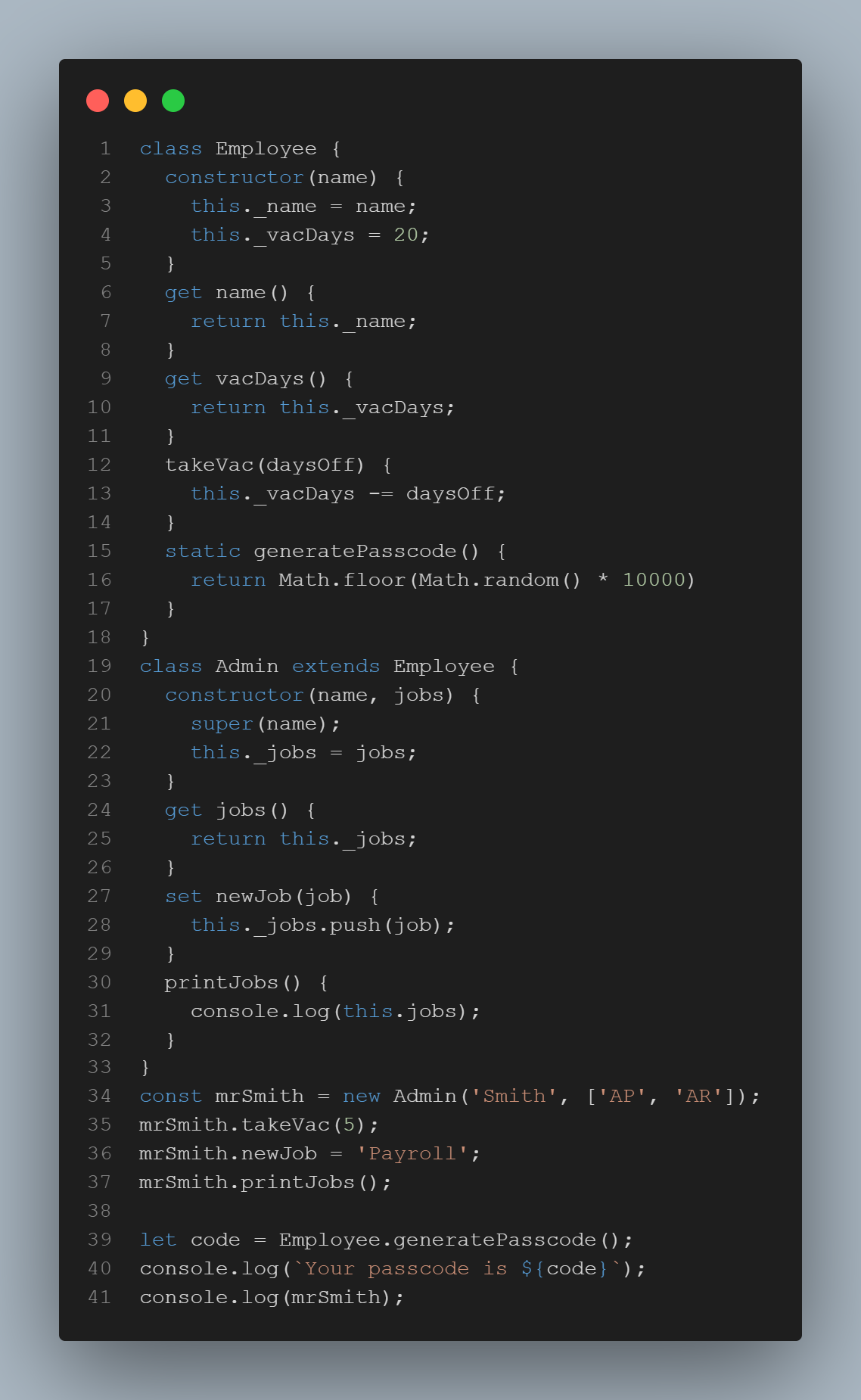
Modules
Each of the two JavaScript runtime environments has a preferred module implementation:
- With the browser: use ES6 import
and export
- With Node: use module.exports
and require()
Implementing modules in the browser
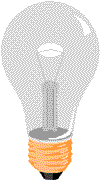
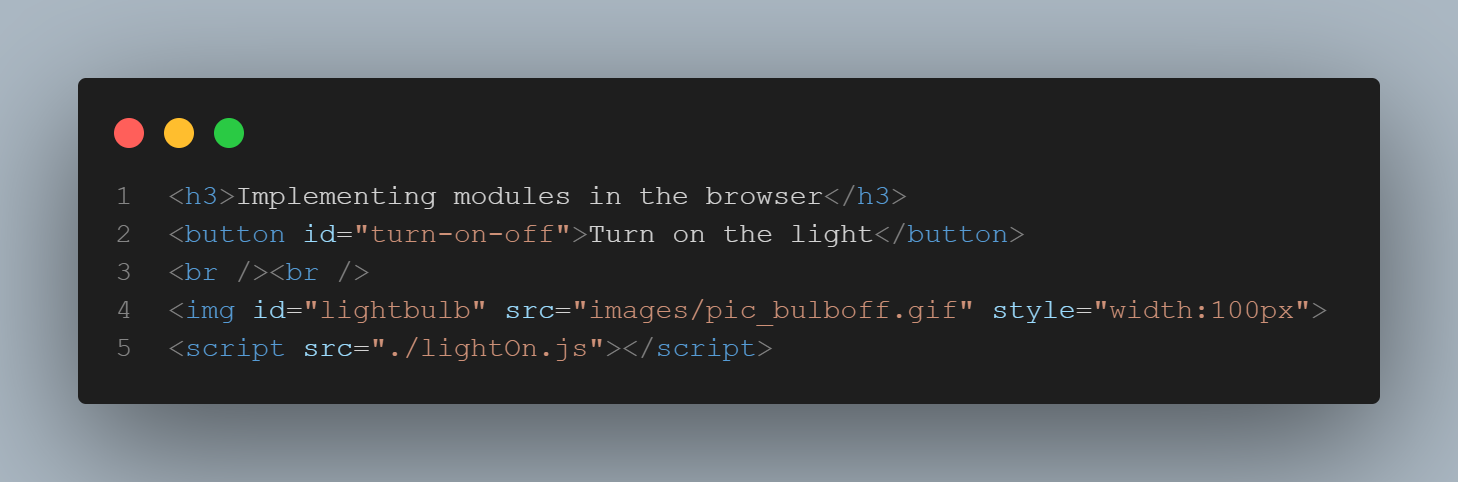
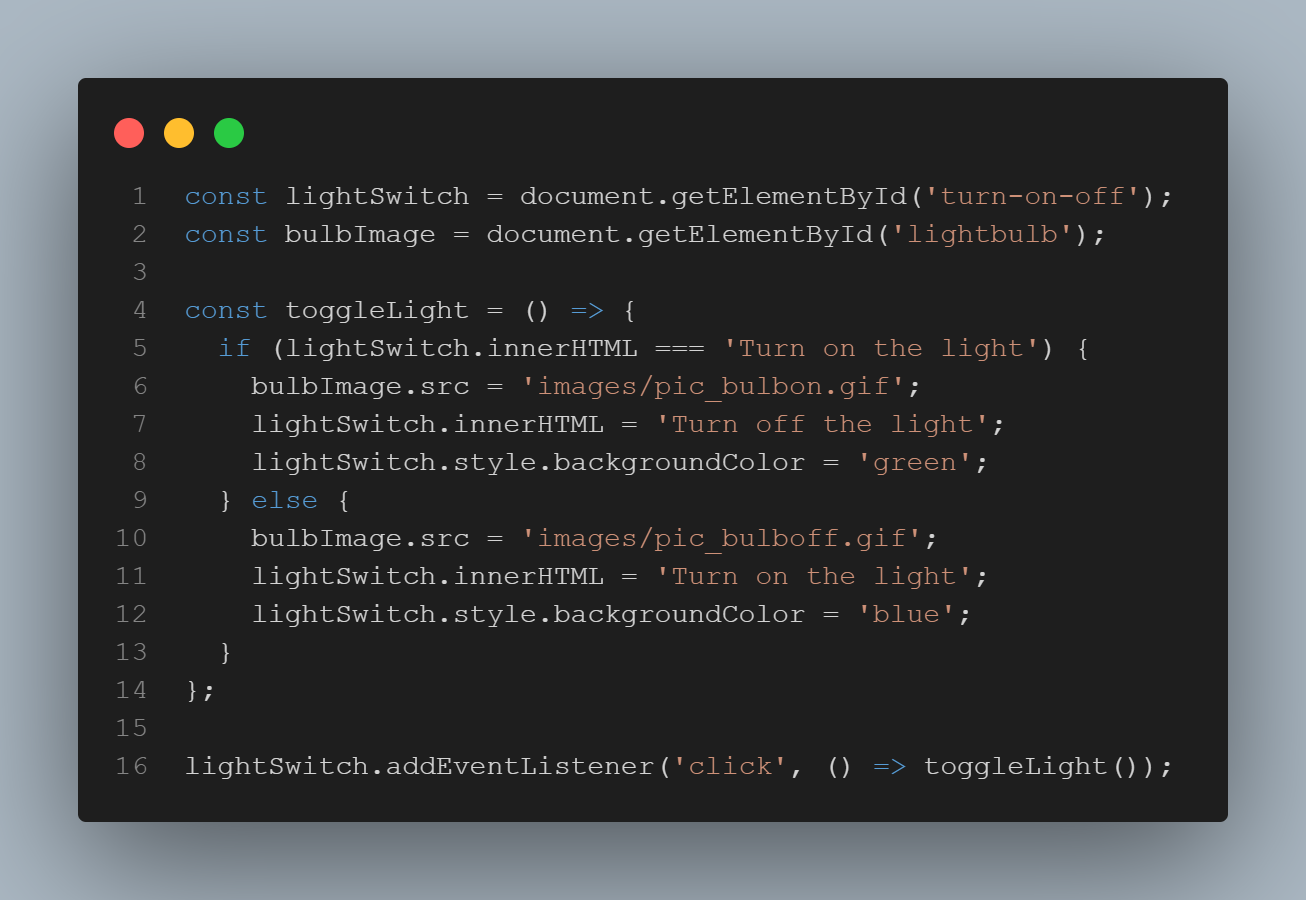
Click the picture if you are bored
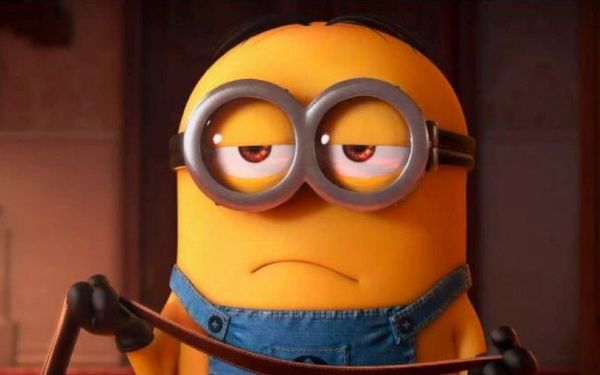

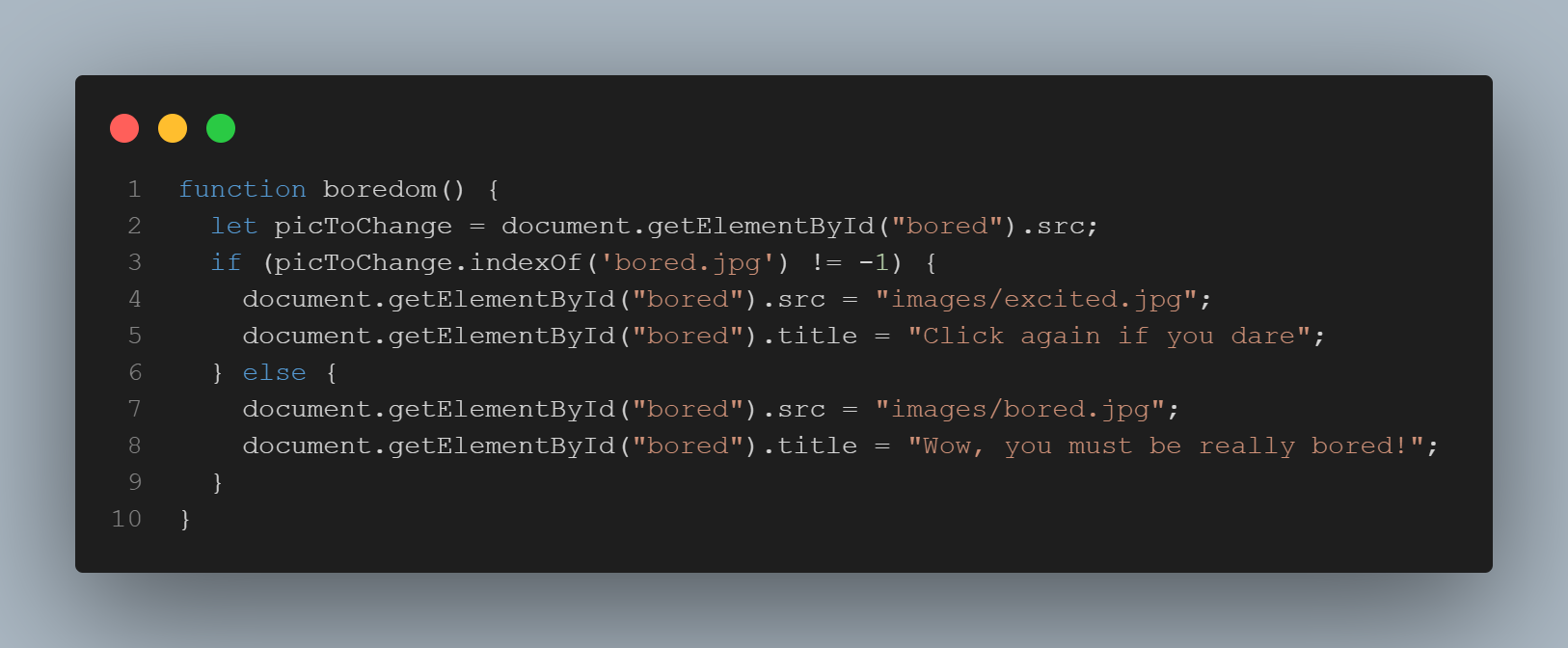
Exporting Modules
Named exports (using function declaration syntax)
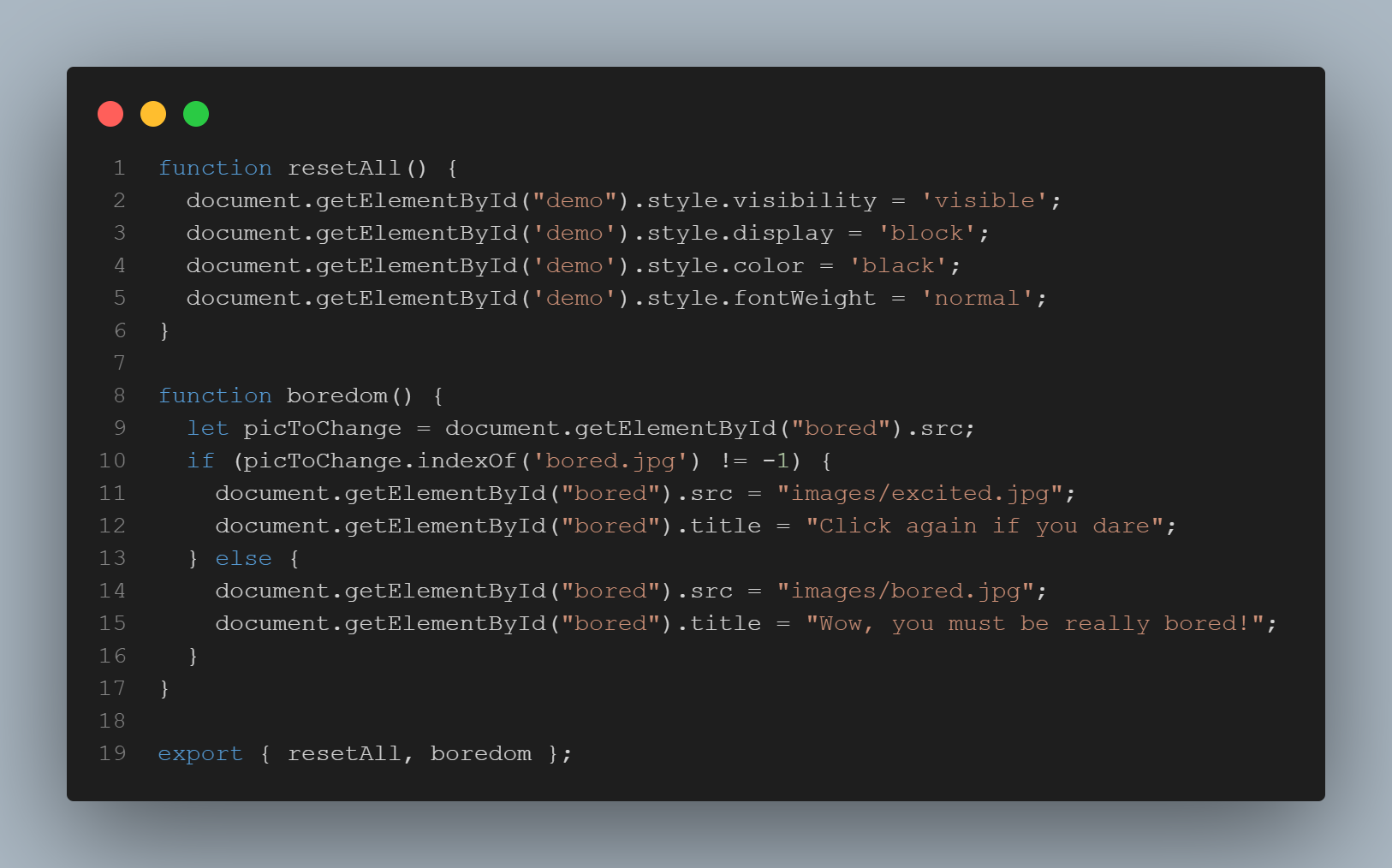
Inline named exports (using arrow function syntax)
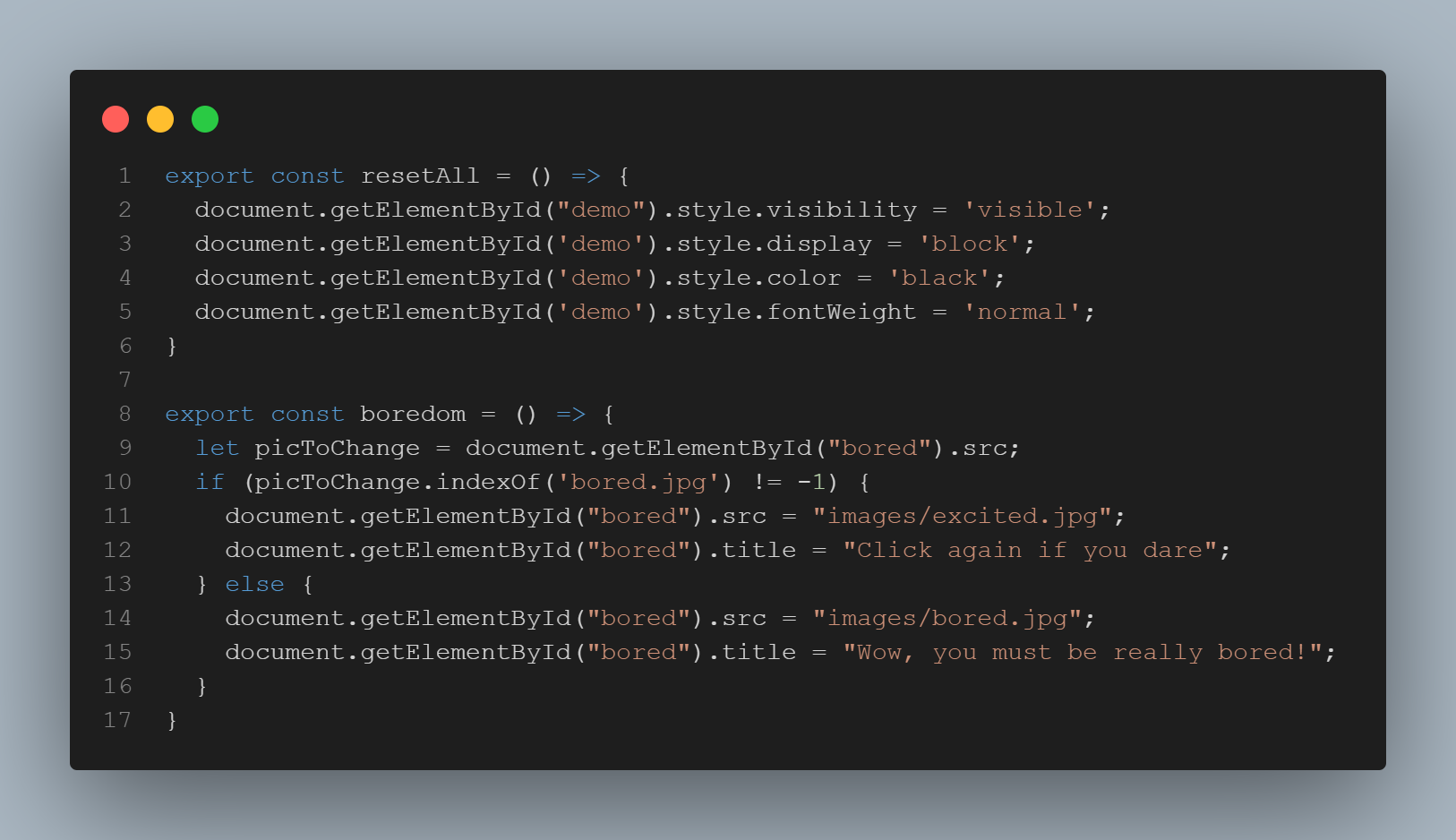
Default exports
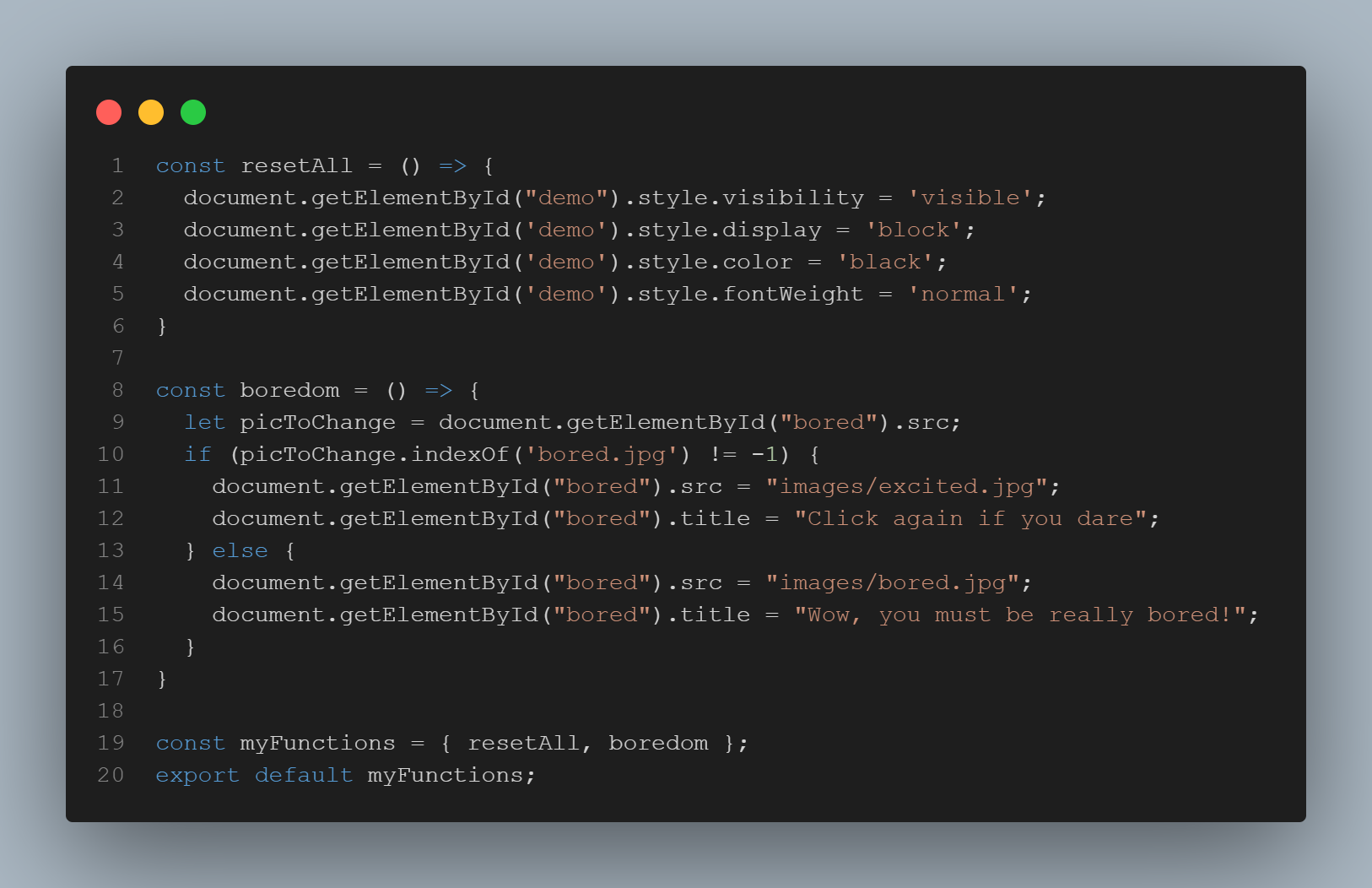
Importing Modules
Named imports (used with named exports or inline named exports)
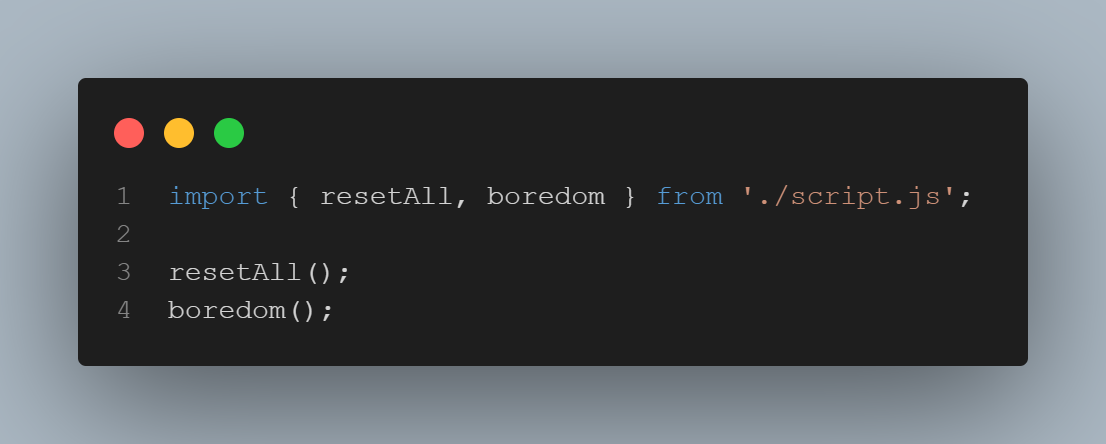
Default imports
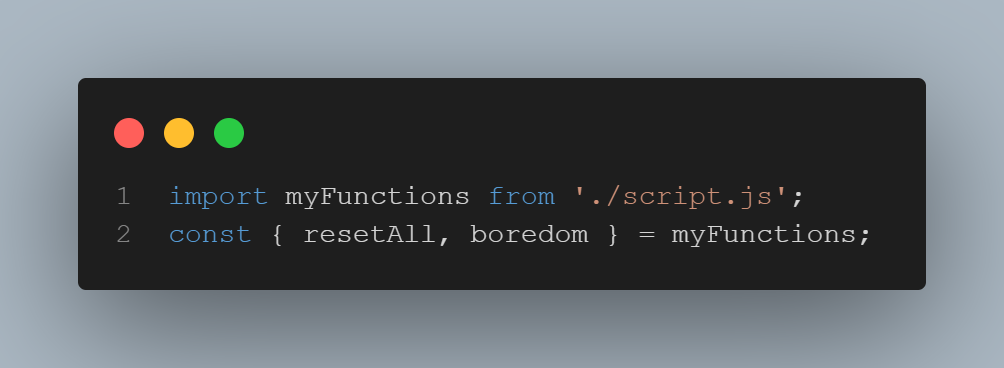
Renamed imports
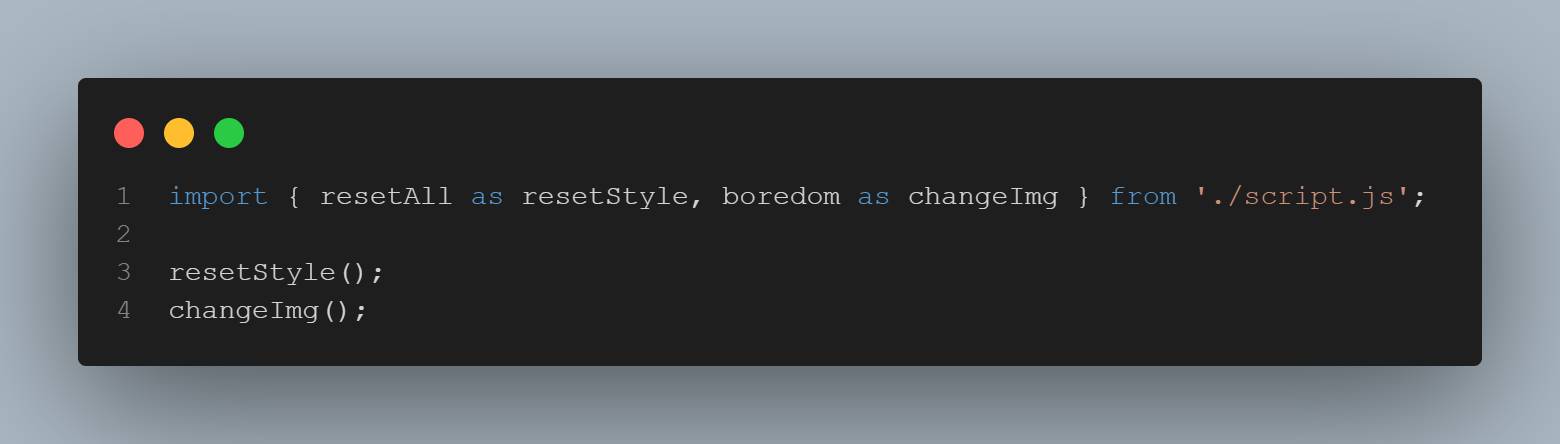
Importing a module in HTML using type="module"
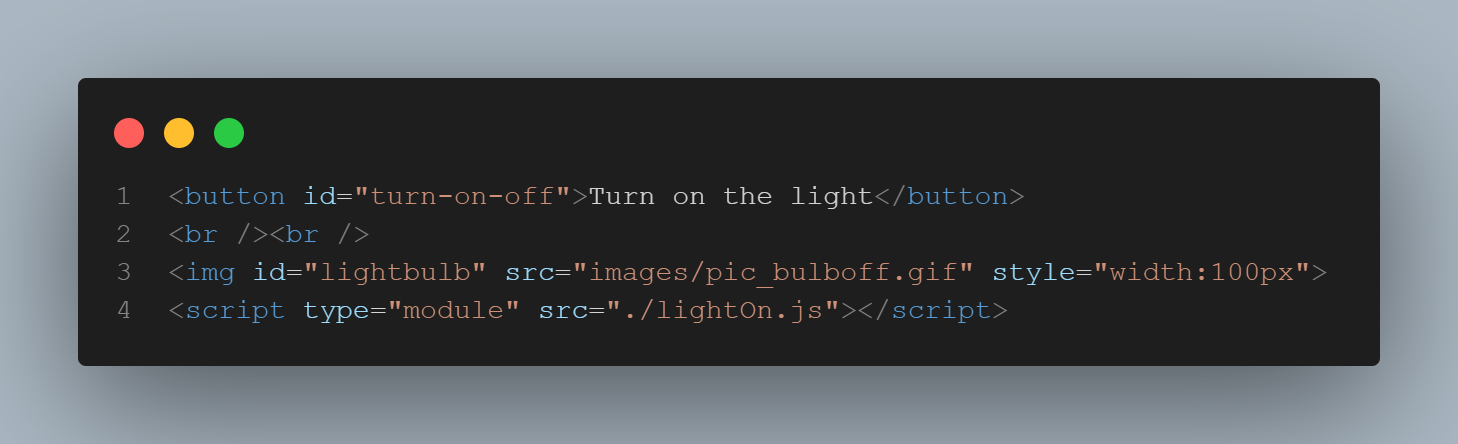
Summary
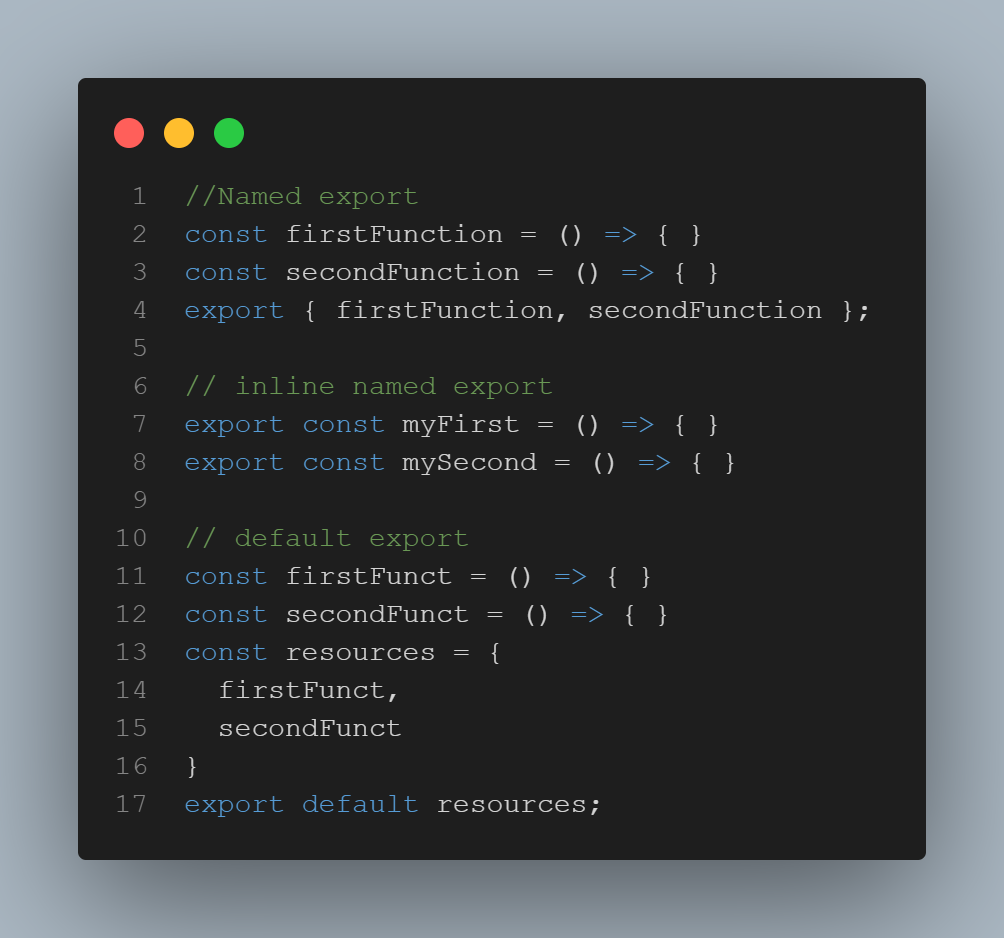
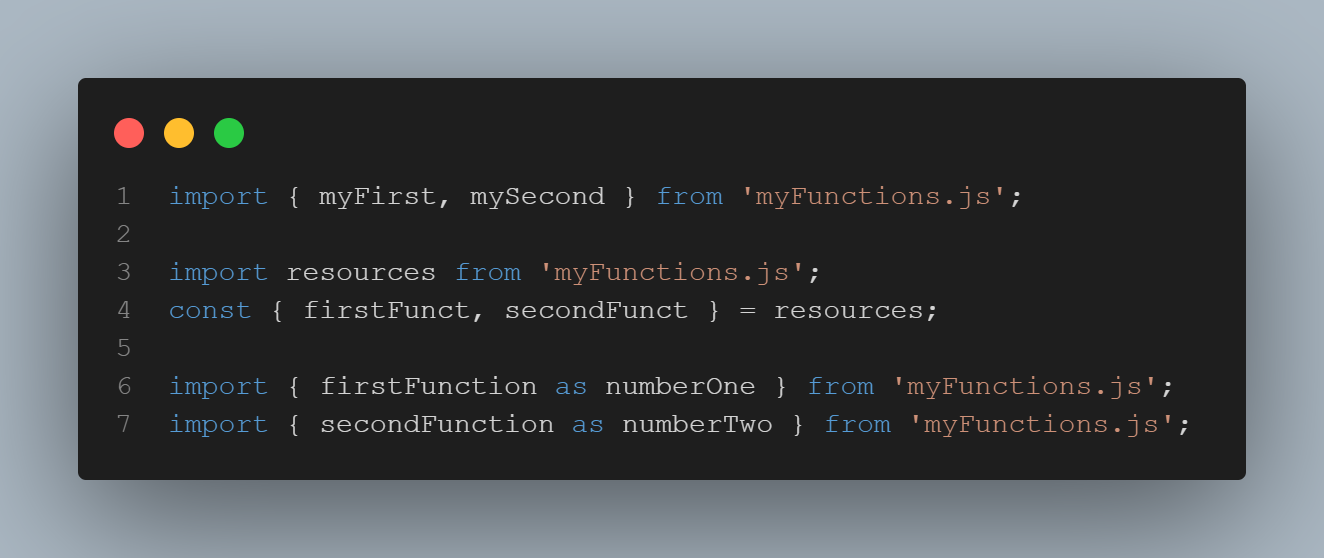